What is HTTP?
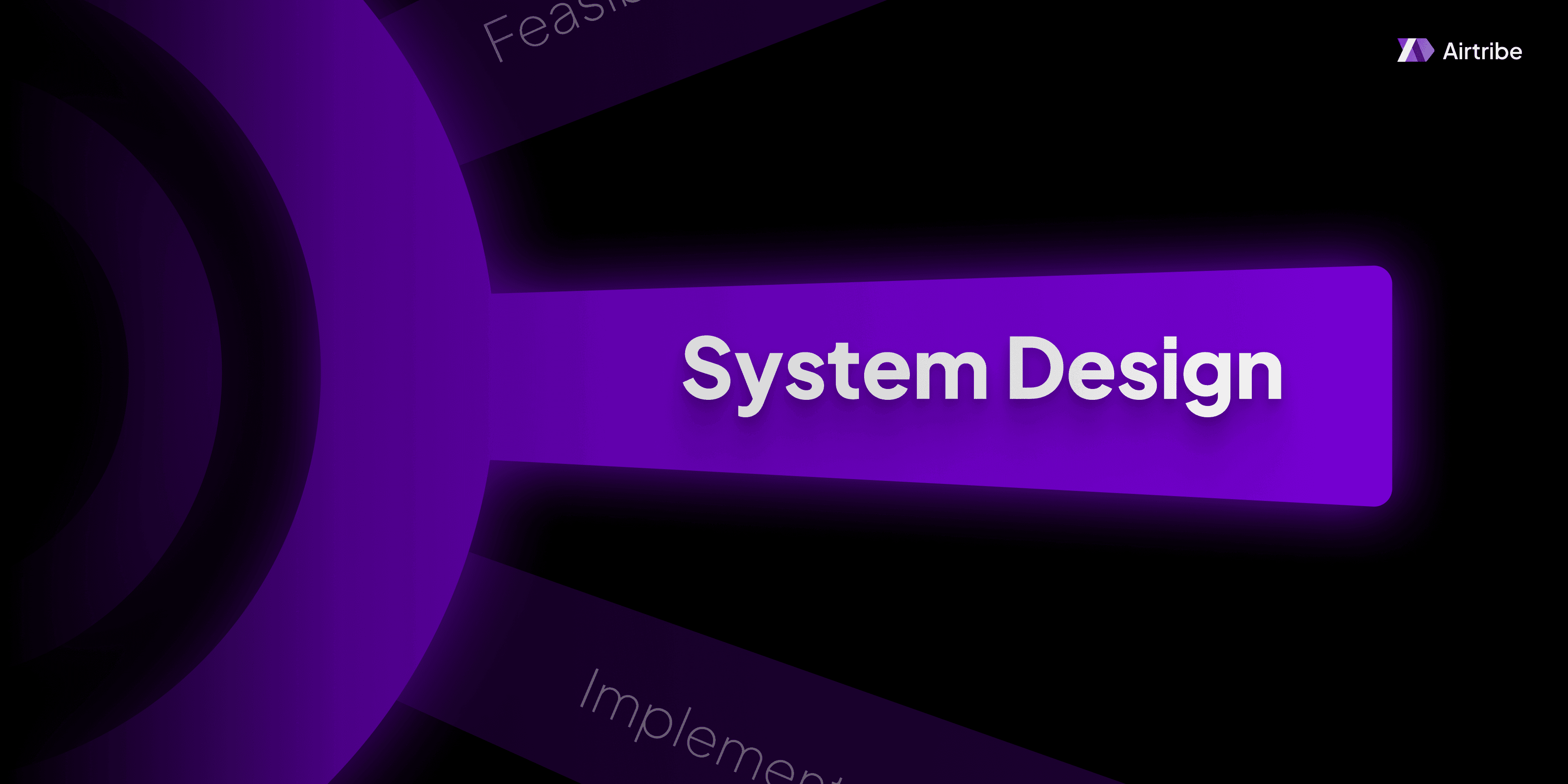
Understanding HTTP
HTTP, or Hypertext Transfer Protocol, serves as the foundation of any data exchange on the Web. It is a protocol used for transmitting hypertext documents, such as web pages. It was developed in the early 1990s by Tim Berners-Lee, the creator of the World Wide Web. HTTP is an application layer protocol designed within the Internet protocol suite.
Core Concepts and Theory
HTTP is a client-server protocol, where a client (usually a web browser) sends a request to a server, and the server responds. It follows a request-response model, which is stateless by nature. Let's break down these core concepts:
Request-Response Model
- Client: Initiates the communication by sending an HTTP request to the server.
- Server: Listens for incoming requests and responds with the requested resources.
- Statelessness: Each HTTP request from a client to server is independent. The server does not remember previous requests. This necessitates the use of cookies or sessions to maintain stateful information.
Structure of HTTP
HTTP communication consists of messages in plain text that are readable by humans. Each message has a specific structure:
- Request Message: Composed of a request line (e.g., GET /index.html HTTP/1.1), headers (providing metadata), and an optional body (containing data).
- Response Message: Composed of a status line (e.g., HTTP/1.1 200 OK), headers, and an optional body.
Methods
HTTP defines several methods indicating the desired action to be performed on a resource:
- GET: Requests data from a specified resource.
- POST: Sends data to a server to create/update a resource.
- PUT: Updates a resource.
- DELETE: Removes a resource.
- HEAD: Similar to GET but without the response body, useful for checking resource meta-information.
Status Codes
HTTP status codes are issued by a server in response to a client's request. They indicate the result of the request:
Status Code | Description |
---|---|
200 | OK |
301 | Moved Permanently |
404 | Not Found |
500 | Internal Server Error |
Versions
- HTTP/1.0: Original version with basic functionality.
- HTTP/1.1: Introduced persistent connections and more cache control mechanisms.
- HTTP/2: Enhanced performance with multiplexing, header compression, and prioritization.
- HTTP/3: Uses QUIC, a transport layer network protocol by Google, for improved performance and security.
Practical Applications
HTTP is integral to web technologies and is used for:
- Web Browsing: All web interactions between browsers and servers rely on HTTP.
- API Requests: Utilizes HTTP to enable communication between different software applications.
- Media Streaming: HTTP is used in progressive downloads and adaptive streaming technologies.
Code Implementation and Demonstrations
Here is a simple example of how to make an HTTP GET request using Python's requests
library:
import requests
# Making a GET request
response = requests.get('https://api.example.com/data')
# Checking the status code of the response
if response.status_code == 200:
print("Request was successful.")
print("Response content:", response.content)
else:
print(f"Failed to retrieve data. Status code: {response.status_code}")
Additional Resources and References
- RFC 2616: Hypertext Transfer Protocol -- HTTP/1.1
- Mozilla Developer Network (MDN) Web Docs: HTTP Overview
- HTTP/2 RFC 7540
- Learning HTTP on W3Schools
Understanding HTTP lays the groundwork for comprehending how web resources communicate and function, making it a crucial component in system design for web applications. The protocol continues to evolve, offering more efficient and secure communication methods as seen in the transition from HTTP/1.0 to HTTP/3.