What is a Message Queue?
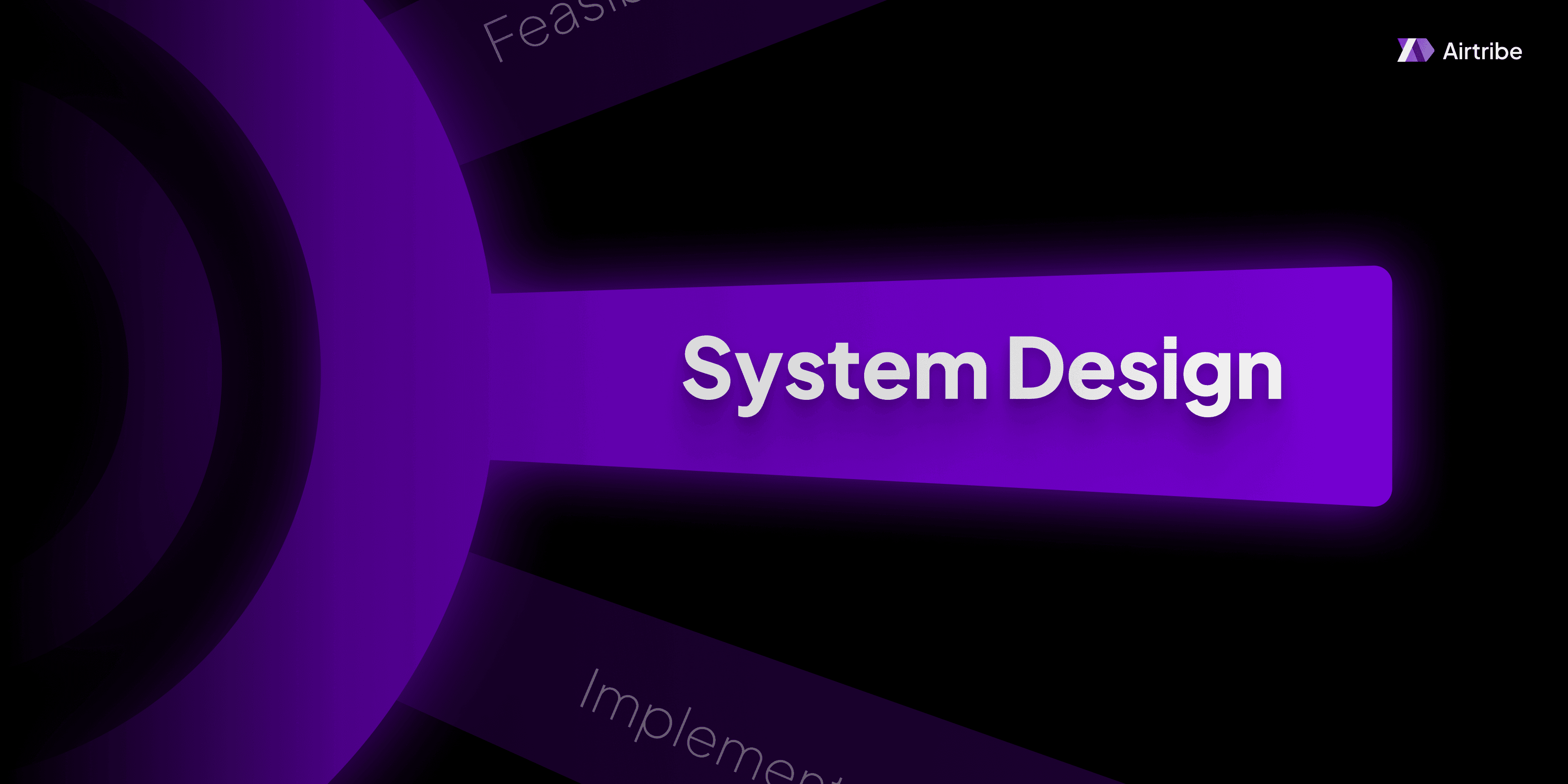
Understanding Message Queues
Message queues are integral components in system design, enabling asynchronous communication between distributed systems and services. This concept is pivotal in building scalable, reliable, and resilient applications, particularly in environments where systems need to communicate across different network nodes.
Core Concepts and Theory
What is a Message Queue?
A message queue is a form of asynchronous service-to-service communication used in serverless and microservices architectures. Messages are stored in a queue until they are processed and removed from the queue. This decouples the sender and receiver, allowing each to function independently.
Key Characteristics of Message Queues
- Asynchronous Communication: Message queues enable non-blocking communication, allowing sender components to continue processing without waiting for a response.
- Decoupling: Systems are loosely coupled as the message sender and receiver interact indirectly.
- Scalability: By allowing asynchronous processing, message queues can handle varying loads more gracefully.
- Reliability: In scenarios where components need to be fault-tolerant, message queues can persist messages until they are successfully processed.
Components of Message Queues
- Producer: The component or application that sends a message to the queue.
- Consumer: The component or application that receives messages from the queue.
- Message Broker: The system responsible for ensuring messages are correctly routed from producers to consumers.
How Does a Message Queue Work?
- Message Creation: A producer creates and sends a message to a queue.
- Message Storage: The message is stored in the queue until a consumer is ready to process it.
- Message Consumption: A consumer retrieves and processes the message. After processing, the message is typically removed from the queue.
Types of Messaging
- Point-to-Point Messaging: Each message in the queue is consumed by a single consumer.
- Publish-Subscribe Messaging: Messages are broadcast to all subscribers. Each subscriber maintains its own copy of the message.
Practical Applications
Use Cases in System Design
- Load Leveling: Managing workloads that vary in intensity by queuing tasks.
- Task Scheduling: Queuing tasks to be completed at a later time.
- Decoupled Microservices Communication: Enabling distinct microservices to communicate asynchronously.
- Event Sourcing and CQRS: Using messages to maintain a log of events and commands in an application.
Popular Message Queue Implementations
- RabbitMQ: Known for its reliability and support for messaging protocols.
- Apache Kafka: Optimized for handling high-throughput and distributed stream processing.
- Amazon SQS: Fully managed message queuing service by AWS, offering simplicity and scalability.
Code Implementation and Demonstrations
Simple Example Using RabbitMQ
import pika
# Establish a connection to RabbitMQ server
connection = pika.BlockingConnection(pika.ConnectionParameters('localhost'))
channel = connection.channel()
# Declare a queue
channel.queue_declare(queue='hello')
# Send a message
channel.basic_publish(exchange='',
routing_key='hello',
body='Hello World!')
print(" [x] Sent 'Hello World!'")
# Close the connection
connection.close()
Consuming Messages
import pika
connection = pika.BlockingConnection(pika.ConnectionParameters('localhost'))
channel = connection.channel()
# Declare a queue
channel.queue_declare(queue='hello')
# Define a callback function to process messages
def callback(ch, method, properties, body):
print(f" [x] Received {body}")
# Start consuming
channel.basic_consume(queue='hello',
on_message_callback=callback,
auto_ack=True)
print(' [*] Waiting for messages. To exit press CTRL+C')
channel.start_consuming()
Comparison and Analysis
Message Queue vs Other Communication Models
Feature | Message Queue | Direct Request-Response |
---|---|---|
Coupling | Loose | Tight |
Scalability | Naturally scalable | Dependent on server capabilities |
Reliability | High (with persistent queues) | Varies |
Real-time | Adequate with real-time capable consumers | Immediate |
Choosing the Right Message Queue
When selecting a message queue, consider factors like message throughput, fault tolerance, ease of management, and integration capabilities.
Additional Resources and References
Message queues are fundamentally transformative in designing systems that require robust asynchronous communication mechanisms. By understanding their core principles, developers gain the ability to create more resilient, scalable, and maintainable systems.