Replacement Policies
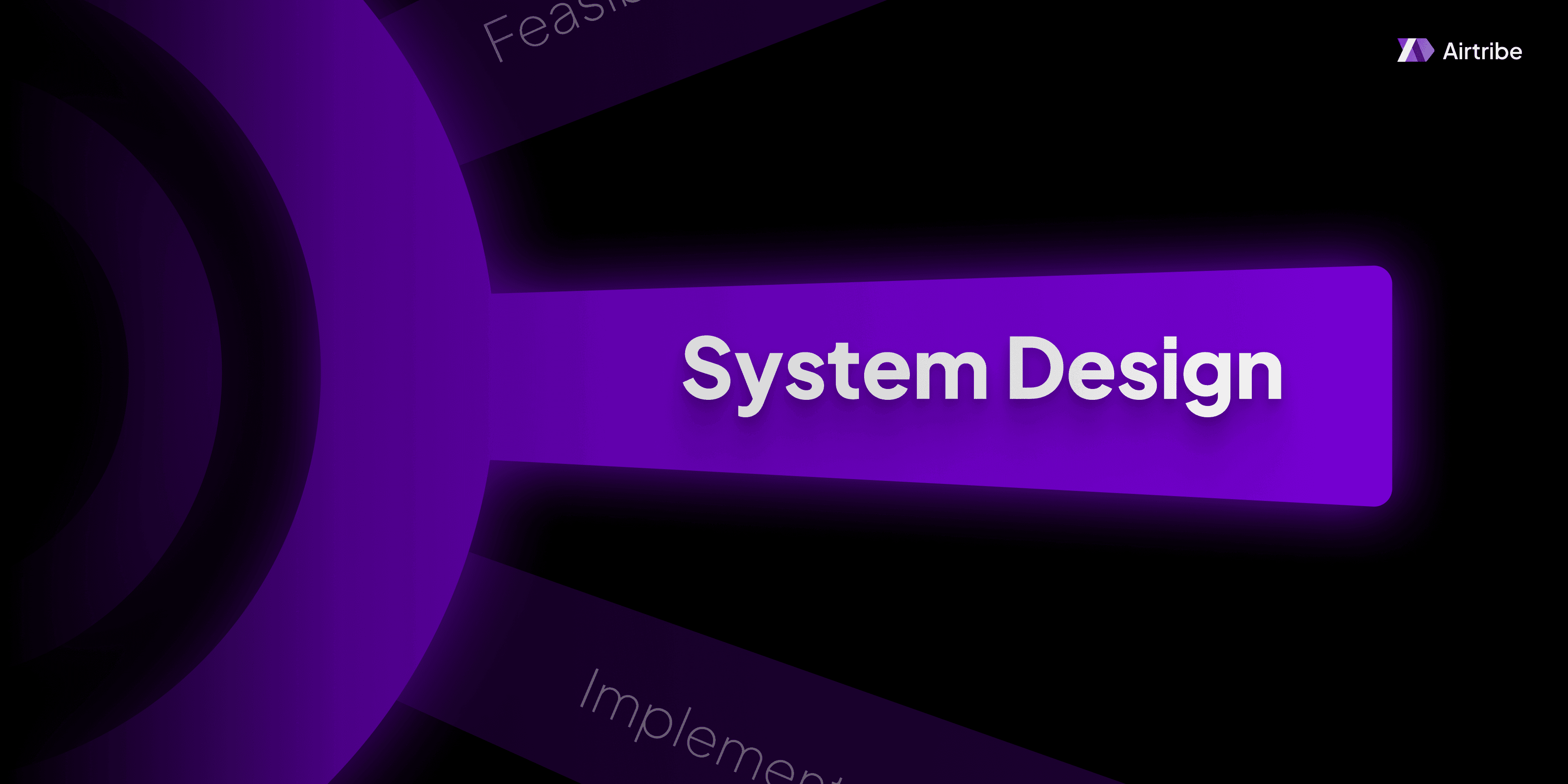
Introduction to Cache Replacement Policies
Caching is a critical concept in system design, aiming to store frequently accessed data in a 'fast-to-retrieve' location to reduce latency and improve performance. However, caches are typically of limited size, necessitating effective cache replacement policies to decide which existing data should be evicted when new data needs to be cached. Replacement policies ensure optimal utilization of caching resources, thereby maintaining system efficiency.
Core Concepts and Theory
Importance of Cache Replacement Policies
Cache replacement policies are essential for maintaining the cache's efficiency by ensuring that the cached data remains relevant to the system's current needs and workloads. The main goal is to prevent cache misses as much as possible and ensure that the most beneficial data is kept in the cache.
Key Metrics
- Hit Rate: The percentage of requests served by the cache.
- Miss Rate: The percentage of requests that the cache cannot fulfill, requiring a fetch from the primary storage.
- Eviction Rate: Frequency of data being removed from the cache to make space for new data.
Popular Replacement Policies
Least Recently Used (LRU): This policy evicts the data that hasn't been accessed for the longest time. It assumes that data accessed recently will likely be used again soon.
First-In-First-Out (FIFO): Evicts the oldest data entry first. While simple, it might not be ideal in all scenarios.
Least Frequently Used (LFU): Evicts data that is accessed least often, which might better represent the long-term usage patterns than LRU.
Random Replacement: Randomly selects a cache entry for eviction. This method can sometimes be surprisingly effective and has minimal overhead.
Most Recently Used (MRU): Contrary to LRU, this approach throws out the most recently accessed data first, based on the premise that it might not be needed again soon.
Practical Applications
Choosing a Replacement Policy
The choice of a cache replacement policy depends on the specific use case and application requirements:
- Web Servers might benefit from LRU or LFU depending on the access patterns and size of data.
- Database Systems often prefer LRU to manage in-memory caching effectively.
- Operating Systems might implement a hybrid approach combining several policies for varied resource management needs.
Implementation Considerations
When implementing a replacement policy, consider:
- Cache Size: Larger caches might handle more complex policies such as LFU or hybrid models.
- Data Volatility: Highly dynamic data can benefit from LRU or MRU.
- Access Patterns: Understanding data access trends can help decide between policies.
Code Implementation and Demonstrations
Let's explore a simple implementation of the LRU cache replacement policy using Python's collections.OrderedDict
.
from collections import OrderedDict
class LRUCache:
def __init__(self, capacity: int):
self.cache = OrderedDict()
self.capacity = capacity
def get(self, key: int) -> int:
if key not in self.cache:
return -1
else:
self.cache.move_to_end(key)
return self.cache[key]
def put(self, key: int, value: int) -> None:
if key in self.cache:
self.cache.move_to_end(key)
self.cache[key] = value
if len(self.cache) > self.capacity:
self.cache.popitem(last=False)
# Example usage
lru = LRUCache(3)
lru.put(1, 1)
lru.put(2, 2)
lru.get(1) # Returns 1
lru.put(3, 3)
lru.put(4, 4)
lru.get(2) # Returns -1 (evicted)
Comparison and Analysis
LRU vs. LFU vs. FIFO
- LRU: Better suited for read-heavy applications where recent data is likely required.
- LFU: Ideal for environments with predictable access patterns and less dynamic data.
- FIFO: Can be beneficial for systems focusing on simplicity and low overhead.
Policy | Use Case | Pros | Cons |
---|---|---|---|
LRU | Web Servers, Database Systems | Simple, intuitive | Might not reflect actual usage frequency |
LFU | Cache for APIs, Data Centers | Reflects access frequency | Higher complexity, requires counting accesses |
FIFO | Simple buffer management | Simple, predictable | May lead to suboptimal caching decisions |
Additional Resources and References
- Cache Replacement Policies by Carnegie Mellon University
- Operating Systems: Three Easy Pieces - Chapter on Caching
- LRU Cache Implementation Guide on GeeksforGeeks
Understanding and selecting the right cache replacement policy is crucial to optimizing system performance and resource management effectively. By examining access patterns, and system requirements, and implementing the appropriate strategies, systems can maintain efficient caching behavior.