Process Program and Thread
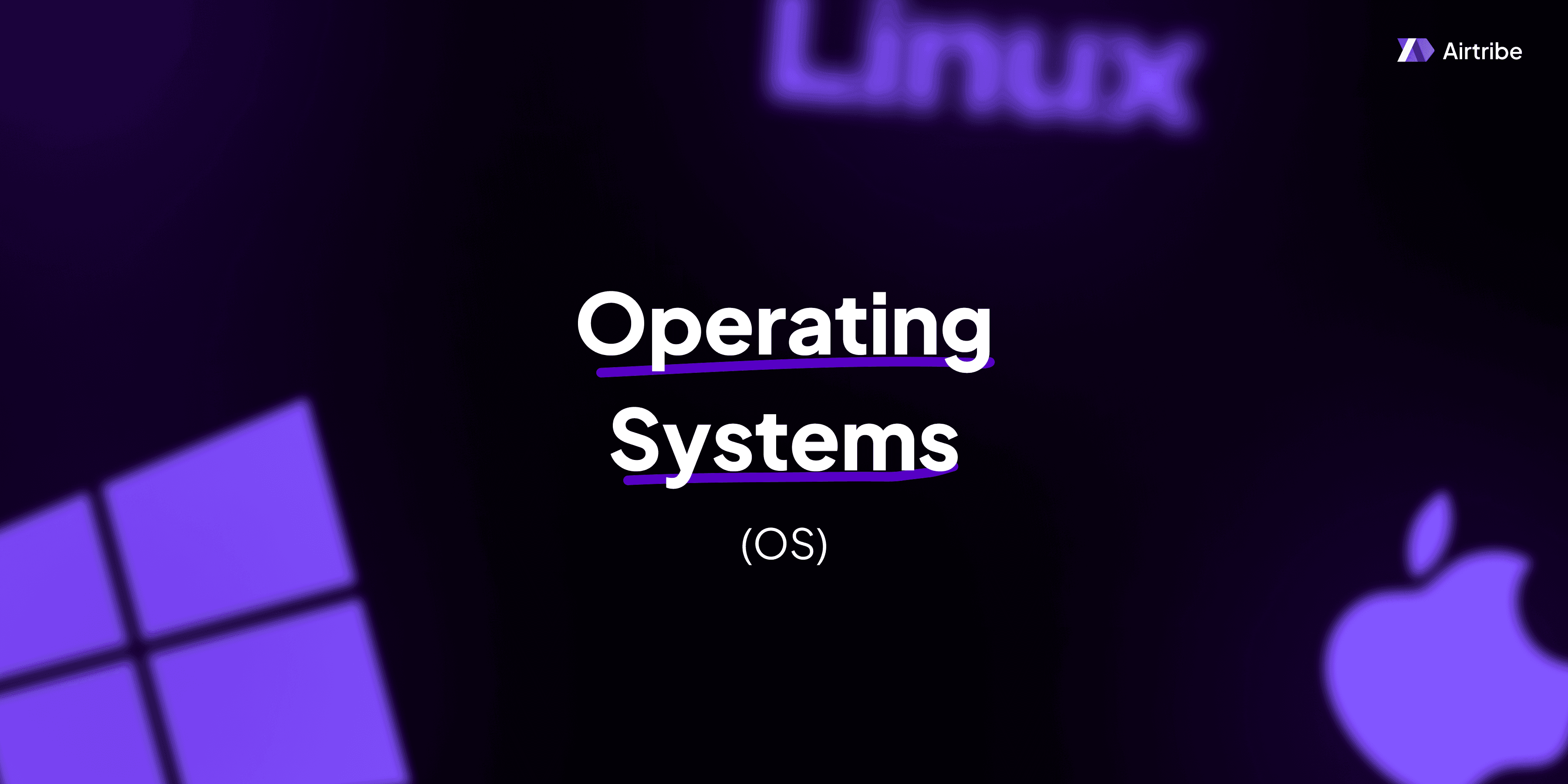
Understanding Processes, Programs, and Threads in Operating Systems
Operating systems (OS) are integral to computer functionality, acting as intermediaries between a user's application and the hardware. Among the most fundamental concepts within operating systems are processes, programs, and threads. Each plays a critical role in how software is executed and managed by the OS. This article provides a comprehensive look at these concepts, explaining their differences, interrelations, and significance in computing.
Core Concepts and Theory
Programs
A program is a static set of instructions that resides on disk and is intended to perform a specific task or solve a particular problem. It is essentially the code written and compiled by developers to execute a sequence of actions. Note that a program by itself does not perform any activity until it is executed or loaded into memory.
- Characteristics:
- Static: Stored on the disk till execution.
- Comprised of Code: Includes binaries and libraries.
Processes
A process is an instance of a program in execution. When a program is run, it becomes a process that can be viewed as the active state. It encapsulates the program's code and its current activity. The process is fundamental to resource management and execution in operating systems.
Key Components:
- Text Segment: The compiled code of the program.
- Data Segment: Contains global and static variables.
- Heap: Dynamically allocated memory during runtime.
- Stack: Holds local variables and function call information.
- Program Counter: Indicates where a process is in its execution sequence.
- Process Control Block (PCB): Contains process-related information like the process state, process ID, scheduling information, etc.
States of a Process:
- New: Process is being created.
- Ready: Waiting to be assigned to a processor.
- Running: Instructions being executed.
- Waiting: Process is waiting for some event to occur.
- Terminated: Process has finished execution.
Threads
A thread is often described as the smallest sequence of programmed instructions that can be managed independently by the operating system's scheduler. Threads are part of a process, and multiple threads can exist within one process, sharing resources but capable of executing concurrently.
Advantages:
- Responsiveness: Allows a program to continue running even if part of it is blocked or is performing a lengthy operation.
- Resource Sharing: Shares the memory and resources of the process to which it belongs.
- Economical: It is more economical to create and manage threads than processes.
Types of Threads:
- User Threads: Managed by the user and typically do not require kernel support.
- Kernel Threads: Managed directly by the operating system.
Practical Applications
Understanding processes and threads is crucial for:
Efficient Resource Management: Each process or thread requires resources such as memory and CPU time, and effective allocation can lead to improved application performance.
Concurrency: Programming using threads allows multiple operations to occur simultaneously, improving application efficiency and responsiveness.
Multitasking: Operating systems leverage processes and threads to handle multiple applications or operations at the same time.
Code Implementation and Demonstrations
Below is a simple Python demonstration using multithreading:
import threading
def print_numbers():
for i in range(10):
print(f"Number: {i}")
def print_letters():
for c in 'abcdefghij':
print(f"Letter: {c}")
# Creating threads
thread1 = threading.Thread(target=print_numbers)
thread2 = threading.Thread(target=print_letters)
# Starting threads
thread1.start()
thread2.start()
# Wait until both threads complete
thread1.join()
thread2.join()
print("Finished executing threads!")
Comparison and Analysis
Aspect | Program | Process | Thread |
---|---|---|---|
Definition | Static code on disk | Instance of program execution | Smallest unit of a process |
Resource Usage | Does not use resources | Has separate memory and resources | Shares resources within a process |
Inter-communication | Not applicable | Complex and resource-intensive | Shared memory space makes it easier |
Creation Overhead | Compiled and stored | Heavy, due to separate resource requirements | Light, shares resources of process |
Additional Resources and References
Books:
- "Operating System Concepts" by Abraham Silberschatz
- "Modern Operating Systems" by Andrew S. Tanenbaum
Online Articles:
Documentation:
Understanding the distinction and relationships between programs, processes, and threads is essential for delving into more advanced topics in operating systems. This foundational knowledge significantly contributes to efficient software design and the capability of multitasking within an operating environment.