Page Fault
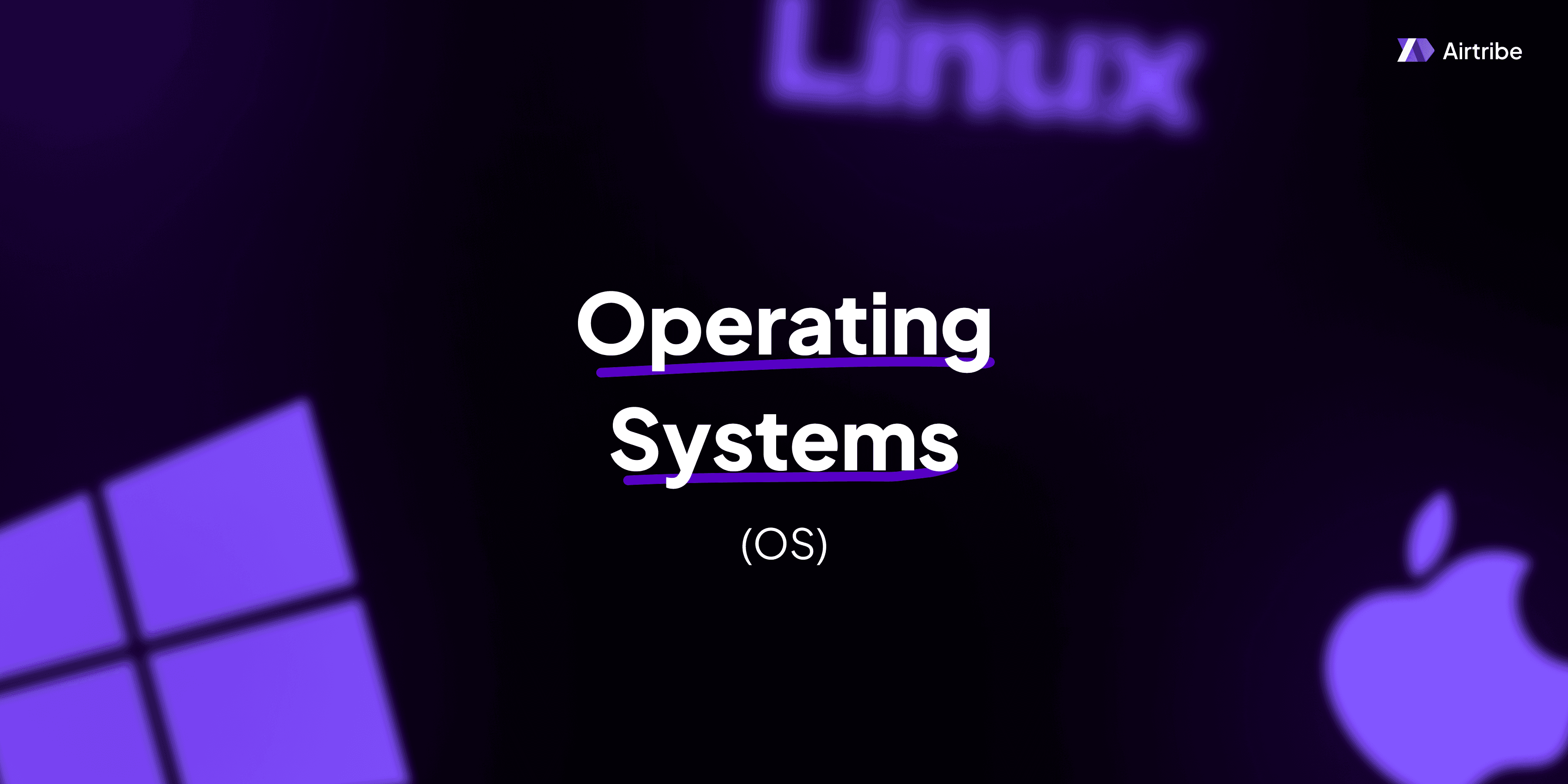
Understanding Page Faults in Memory Management
Memory management is a critical component of operating systems that ensures efficient use and allocation of memory resources to various processes. One significant concept in this domain is that of page faults. This article explores the fundamental aspects of page faults, their occurrence, handling mechanisms, and their implications in memory management.
Core Concepts and Theory
What is a Page Fault?
A page fault occurs when a running program accesses a portion of memory that is not currently in physical RAM. This typically happens in systems that utilize virtual memory, a technique that extends the apparent memory available to programs by using disk storage to simulate extra RAM.
Virtual Memory and Paging
In systems with virtual memory, memory is divided into blocks of equal size called pages. The physical memory (RAM) is subdivided into frames that are of the same size as pages. When a process executes, its pages are loaded into any available frames, creating a mapping between the virtual and physical address spaces. A page table maintains this mapping, allowing the operating system (OS) to translate virtual addresses to physical addresses efficiently.
Causes of Page Faults
Page faults can occur due to several reasons:
- Page Not in Memory: The requested page is not in RAM, requiring it to be loaded from disk (secondary storage).
- Page Protection Violation: The process tries to access a page without the appropriate permissions.
- Page Table Entry Invalid: The page table doesn't contain a valid entry for the requested page due to initial loading requirements or data corruption.
Handling Page Faults
When a page fault occurs, the operating system handles the situation through a series of steps:
- Determine the Cause: Identify why the page fault occurred—whether the page was not present or was due to a protection violation.
- Resolve the Fault:
- If the page is not in memory, select the appropriate page from disk and load it into an available frame in RAM.
- If no frames are available, use a page replacement algorithm to select a victim page to remove from memory.
- Update the Page Table: Adjust the page table to reflect the new state of memory.
- Restart the Process: Reissue the interrupted instruction now that the page is in memory.
Practical Applications
Page Replacement Algorithms
Page replacement algorithms play a crucial role in resolving page faults efficiently. Common algorithms include:
- FIFO (First-In-First-Out): Replaces the oldest page in memory.
- LRU (Least Recently Used): Replaces the page that has not been used for the longest period.
- Optimal Page Replacement: Replaces the page that will not be used for the longest future period (theoretical and mainly used for benchmarking).
Impact on System Performance
Frequent page faults can degrade system performance, leading to a situation known as "thrashing," where the OS spends more time handling page faults than executing actual processes. Minimizing page faults is crucial for efficient memory management and involves:
- Appropriately sizing the physical memory and managing the working set.
- Optimizing the page replacement algorithm based on workload characteristics.
Code Implementation and Demonstrations
Here we present a simple Python implementation to simulate a page replacement using the FIFO algorithm:
def fifo_page_replacement(pages, frame_size):
memory = []
page_faults = 0
for page in pages:
if page not in memory:
page_faults += 1
if len(memory) < frame_size:
memory.append(page)
else:
memory.pop(0)
memory.append(page)
print(f"Page: {page}, Memory: {memory}")
return page_faults
pages = [1, 2, 3, 4, 1, 2, 5, 1, 2, 3, 4, 5]
frame_size = 3
faults = fifo_page_replacement(pages, frame_size)
print(f"Total Page Faults: {faults}")
This script demonstrates the FIFO page replacement strategy and calculates the number of page faults.
Comparison and Analysis
Understanding the trade-offs between various page replacement algorithms is essential for optimizing system performance:
Algorithm | Strengths | Weaknesses |
---|---|---|
FIFO | Simple implementation | May replace frequently used pages |
LRU | Accounts for pages used recently | More complex to implement due to tracking usage |
Optimal | Minimal page faults in theory | Impossible to implement practically |
Additional Resources and References
For further exploration of page faults and advanced memory management topics, the following resources are recommended:
- "Operating System Concepts" by Silberschatz, Galvin - A comprehensive guide to operating system fundamentals.
- Computer Systems: A Programmer's Perspective - Delves into how computer systems execute programs.
- Online Courses and Discussions - Platforms like Coursera, edX, and Stack Overflow to keep up with the latest practices and community advice.
With a thorough understanding of page faults and memory management strategies, software development engineers can design more efficient systems and troubleshoot performance bottlenecks effectively.