Batch OS
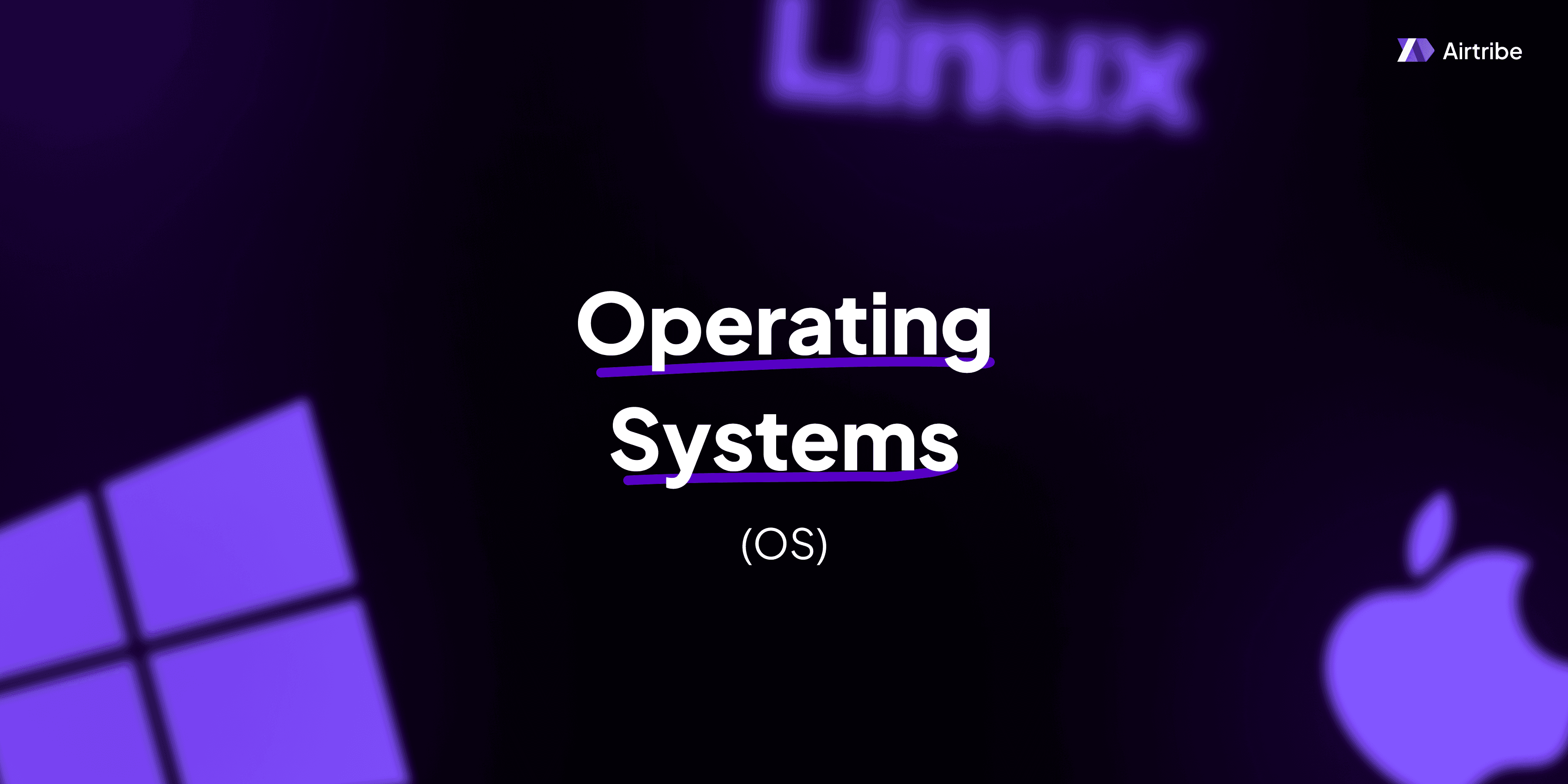
Introduction to Batch Operating Systems
The evolution of operating systems marks a fascinating journey through the history of computing. Among the milestones of this technological trek is the Batch Operating System, which was one of the first types of operating systems developed. Batch OS played a pivotal role during the early days of computing, providing a foundation for more sophisticated operating systems that followed.
Core Concepts and Theory
Definition and Overview
A Batch Operating System is a type of operating system that processes batches of jobs without any direct interaction from the user. Jobs with similar requirements are batched together and executed sequentially. This approach contrasts with modern interactive and time-sharing systems.
Historical Context
Batch processing systems emerged in the 1950s and 1960s when computers were enormously expensive and time-consuming to operate. They were developed to make the use of computer systems more efficient and economical.
How Batch OS Works
- Job Scheduling: Jobs are submitted to a central place, often on punch cards, tapes, or disks. The system automatically schedules these jobs.
- Execution: The operating system executes jobs one after another, sequentially processing batches, which reduces the idle time of the CPU.
- No User Interaction: Users do not interact directly with the system during job execution. They submit jobs and collect results later, allowing for efficient use of computer resources.
Advantages
- Efficiency: By batching similar jobs, Batch OS minimizes CPU idle time and maximizes throughput.
- Automation: It automates the job scheduling and execution process, reducing the need for manual intervention.
Limitations
- Lack of Interaction: Users cannot interact with their jobs during execution, making real-time processing impossible.
- Error Handling: Debugging is cumbersome because errors are often detected only after job completion.
Practical Applications
Batch Operating Systems are mostly historical, but they laid the groundwork for modern operating environments. They are still relevant in certain scenarios:
- Transaction Processing: Used in financial systems for banking transactions.
- Payroll Systems: Handles large volumes of payroll data on a scheduled basis.
- Data Processing: Used in scenarios where a large amount of data needs to be processed without interruption, like data warehousing.
Code Implementation and Demonstrations
While modern systems do not require direct implementation of a Batch OS, understanding their mechanisms can provide insights into job scheduling and automation. For educational purposes, Python can simulate a simple batch processing system:
class Job:
def __init__(self, name, duration):
self.name = name
self.duration = duration
def execute(self):
print(f"Executing job: {self.name}")
# Simulating job execution delay
time.sleep(self.duration)
print(f"Job {self.name} completed")
def batch_process(jobs):
for job in jobs:
job.execute()
import time
# Sample Jobs
jobs = [Job("Job1", 2), Job("Job2", 1), Job("Job3", 3)]
batch_process(jobs)
This code simulates the scheduling and execution of jobs in a batch processing system, each job represented by a simple class with an execution method.
Comparison and Analysis
To truly appreciate the impact of Batch OS, it's useful to compare it with later developments:
Feature | Batch OS | Time-Sharing OS | Modern Interactive OS |
---|---|---|---|
User Interaction | None | Limited | Full |
Processing Efficiency | High | Moderate | High |
Real-Time Processing | No | Yes (Limited) | Yes |
Error Handling | Post Execution | Interactive/Immediate | Real-Time |
This table highlights the trade-offs between different systems, illustrating why Batch OS was suitable at the time and how it paved the way for more interactive systems.
Additional Resources and References
To delve deeper into the history and functioning of Batch Operating Systems and their evolution, consider the following resources:
- Books: "Operating System Concepts" by Abraham Silberschatz, Peter B. Galvin, and Greg Gagne.
- Online Courses: Check platforms like Coursera or edX for courses on operating systems fundamentals.
- Research Papers: IEEE Xplore has papers on historical operating system developments.
Understanding Batch OS provides insight not only into the history of computing but also foundational concepts that influence modern operating systems.