Banker's algorithm
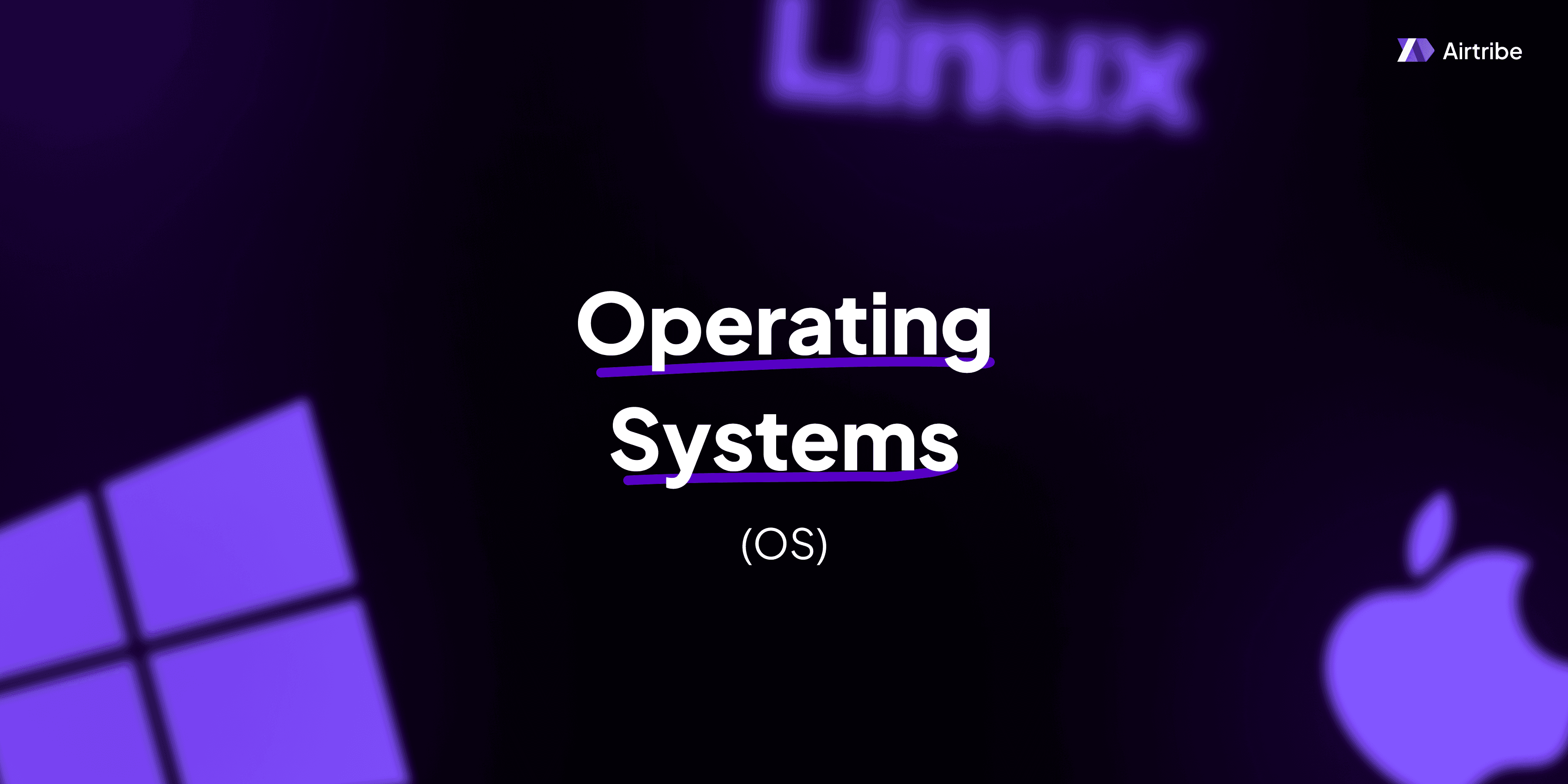
Introduction to Banker's Algorithm
The Banker's Algorithm is a well-established resource allocation and deadlock avoidance method used in operating systems. Named after the way banks manage loan and resource distribution, this algorithm ensures that all system processes get the resources they need without leading the system into a deadlock state. This article explores the core concepts, practical implementation, and analysis of the Banker's Algorithm in the context of process management.
Core Concepts and Theory
Deadlock in Operating Systems
A deadlock occurs when a set of processes are unable to progress because each process is waiting for resources held by the other processes in the set. To manage this, operating systems employ various strategies, one of which is deadlock avoidance.
Resource Allocation
In any operating system, processes require resources like CPU time, memory, and I/O devices to execute. Proper resource allocation ensures system efficiency and stability, crucially avoiding scenarios like deadlocks.
Banker's Algorithm Overview
The Banker's Algorithm was designed by Edsger Dijkstra and functions as a deadlock avoidance algorithm. The algorithm works on the principle of granting a request if and only if it leads the system to a safe state — that is, in a state where all processes can eventually obtain all the resources they require and complete execution.
Definitions in Banker's Algorithm
- Available: A list of available resources of each type.
- Max: The maximum demand of each process.
- Allocation: The current number of resources allocated to each process.
- Need: Remaining resource need for the process, calculated as
Need = Max - Allocation
.
Safe State and Safe Sequence
- Safe State: A state is considered safe if there is a sequence of all processes such that each process can be executed to completion with the currently available resources augmented by the resources held by all the previous processes in the sequence.
- Safe Sequence: An order of processes where each process can be executed without leading to a deadlock.
Practical Applications
When to Use Banker's Algorithm?
The Banker's Algorithm is suitable in environments where:
- The number of processes and resources is static and predetermined.
- Predictability and stability are prioritized over simplicity and speed.
- A higher overhead of computation for deadlock avoidance is acceptable.
It is ideal in systems like mainframes or banking systems where resource allocation is critical, and operations depend on avoiding deadlock scenarios.
Code Implementation and Demonstrations
Here's a simplified implementation of the Banker's Algorithm in Python:
# Function to check if a system is in a safe state
def is_safe(processes, available, max_demand, allocation):
n = len(processes) # Number of processes
m = len(available) # Number of resources
finish = [False] * n
work = available[:]
safe_sequence = []
while len(safe_sequence) < n:
found = False
for i in range(n):
if not finish[i]:
# Check if needs can be met
if all(max_demand[i][j] - allocation[i][j] <= work[j] for j in range(m)):
for j in range(m):
work[j] += allocation[i][j]
safe_sequence.append(processes[i])
finish[i] = True
found = True
break
if not found:
return False, []
return True, safe_sequence
# Example usage
processes = [0, 1, 2, 3]
available = [3, 3, 2]
max_demand = [[7, 5, 3], [3, 2, 2], [9, 0, 2], [2, 2, 2]]
allocation = [[0, 1, 0], [2, 0, 0], [3, 0, 2], [2, 1, 1]]
safe, sequence = is_safe(processes, available, max_demand, allocation)
if safe:
print(f"System is in a safe state. Safe sequence is: {sequence}")
else:
print("System is not in a safe state.")
This code checks if the system is in a safe state based on allocated and available resources.
Comparison and Analysis
Advantages
- Avoids Deadlocks: Proactively checks requests against potential deadlocks.
- Predictable and Systematic: Provides clear pathways to resource allocation strategies.
Disadvantages
- Computational Overhead: Checking every allocation request can be time-consuming.
- Limited Flexibility: Not suitable for dynamic systems with real-time changes in resource allocation.
Comparison with other Techniques
- Deadlock Prevention: Techniques like resource ordering and requiring all resources at once can be less efficient.
- Deadlock Ignorance: Some systems simply choose to ignore the problem altogether (such as many UNIX systems), trading off occasional system stalls for simplicity.
Additional Resources and References
To dive deeper into Banker's Algorithm and related concepts, consider accessing the following resources:
- Operating System Concepts by Abraham Silberschatz, Peter B. Galvin, and Greg Gagne.
- Modern Operating Systems by Andrew S. Tanenbaum.
- Various online coding platforms like GeeksforGeeks and Coding Geeks for practical implementations.
These resources will offer additional insights and examples for understanding the Banker's Algorithm and its role in modern-day operating systems.