B and B+ trees
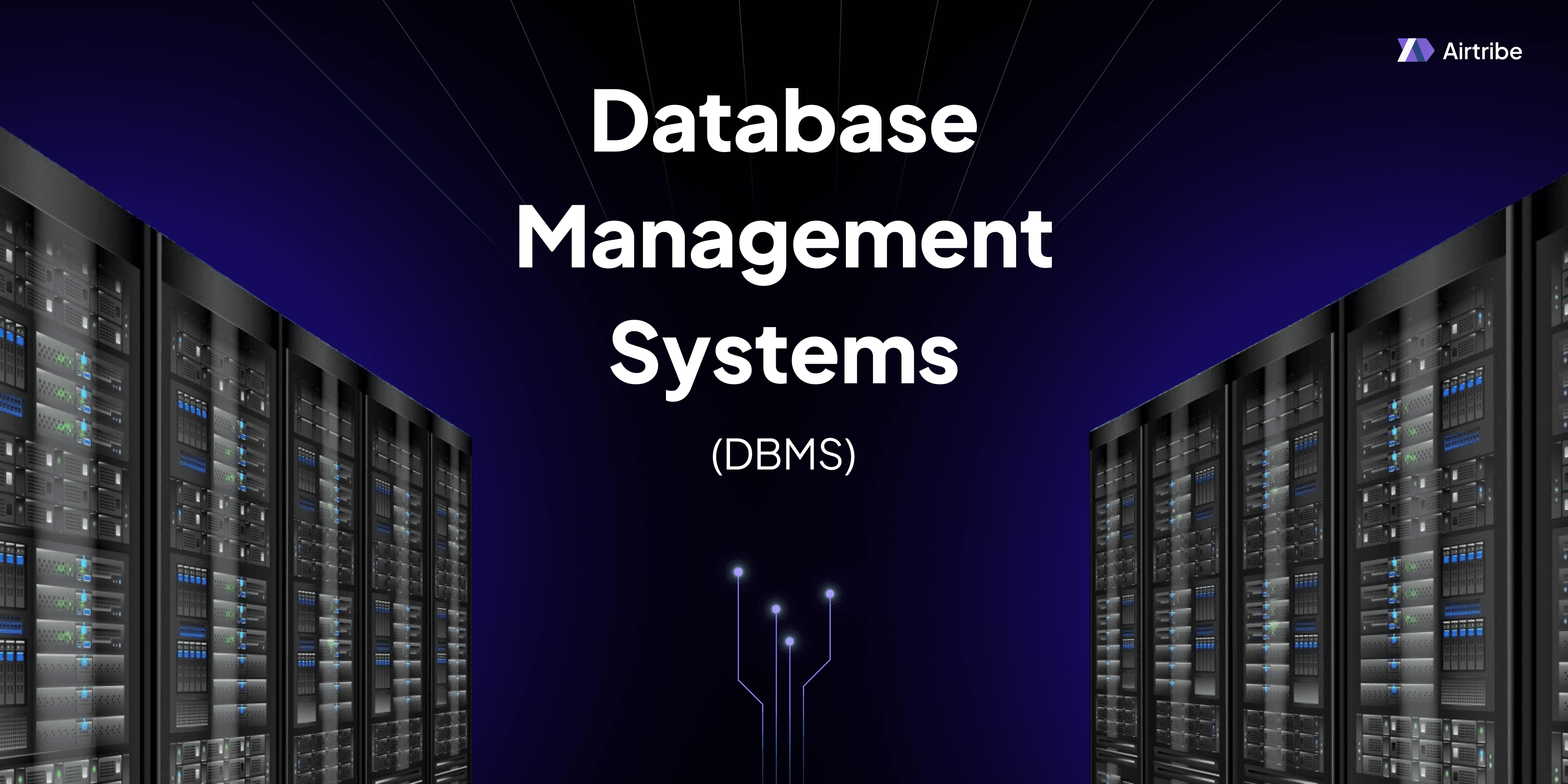
Understanding B and B+ Trees in Database Management Systems
When it comes to optimizing queries in database management systems (DBMS), efficient data retrieval mechanisms are key. B-trees and B+ trees are rooted data structures that play a critical role in indexing database management, which directly influences query optimization. This article delves into the core concepts of these trees, their practical applications, and their implementations.
Core Concepts and Theory
Overview of B-trees
A B-tree is a self-balancing tree data structure that maintains sorted data and allows searches, sequential access, insertions, and deletions in logarithmic time. B-trees are extensively used in database and file systems due to their ability to handle large blocks of data efficiently.
Characteristics of B-trees:
- Balanced Tree: All paths from the root to the leaves are of the same length, ensuring consistent performance.
- Variable Number of Children: Each node contains a variable number of keys and can have more than two children.
- M-way Tree Structure: The B-tree is known as a generalization of binary search trees (BST) by allowing more than two leaves per node.
- Key Order Sorting: Keys within a node are kept in sorted order for efficient search operations.
Overview of B+ Trees
B+ trees are a variation of B-trees and are designed to improve query performance. They are used as indexing structures in DBMS due to their efficiency in reading and maintaining sorted data.
Characteristics of B+ trees:
- Data Store: The internal nodes store keys, while leaf nodes store keys and actual data pointers.
- Dense Indexing: All record pointers are stored at the leaf level, providing a dense index.
- Sequential Navigation: Leaf nodes are linked, enabling easy in-order traversal for range queries.
- Improvements Over B-Tree: Increased fan-out and reduced height, resulting in fewer disk I/O operations.
Practical Applications
The primary application of B-trees and B+ trees is in database indexing. They allow for efficient execution of search queries, such as finding records with specific keys, inserting new records, and deleting existing records, all of which are fundamental database operations.
- Query performance: By minimizing the number of nodes accessed during search, B-trees and B+ trees significantly enhance query processing time compared to sequential scan methods.
- Multi-level Indexing: They support multi-level indexing, which aids in managing very large databases that exceed internal memory.
Code Implementation and Demonstrations
To illustrate the implementation, consider a basic insertion operation in a B+ tree. While the logic is complex due to the need to manage node splits and redistributions, this simplified Python snippet demonstrates the conceptual algorithm:
class BPlusTreeNode:
def __init__(self, t, leaf=False):
self.t = t # Minimum degree (defines the range for number of keys)
self.leaf = leaf # Is true when node is leaf. Otherwise false
self.keys = [] # An array of keys
self.children = [] # An array of child pointers
class BPlusTree:
def __init__(self, t):
self.root = BPlusTreeNode(t, True)
self.t = t # Minimum degree
def insert(self, k):
# Insert logic with node splitting
if len(self.root.keys) == (2 * self.t) - 1:
new_root = BPlusTreeNode(self.t)
new_root.children.append(self.root)
self.split_child(new_root, 0)
self.root = new_root
current = self.root
if current.leaf:
self.insert_leaf(current, k)
else:
while not current.leaf:
idx = self.find_key(current, k)
if len(current.children[idx].keys) == (2 * self.t) - 1:
self.split_child(current, idx)
if k > current.keys[idx]:
idx += 1
current = current.children[idx]
self.insert_leaf(current, k)
def find_key(self, node, k):
idx = 0
while idx < len(node.keys) and k > node.keys[idx]:
idx += 1
return idx
def insert_leaf(self, leaf, k):
leaf.keys.append(k)
leaf.keys.sort()
def split_child(self, parent, idx):
t = self.t
node_to_split = parent.children[idx]
new_node = BPlusTreeNode(t, node_to_split.leaf)
parent.children.insert(idx + 1, new_node)
parent.keys.insert(idx, node_to_split.keys[t - 1])
new_node.keys = node_to_split.keys[t:(2 * t) - 1]
node_to_split.keys = node_to_split.keys[:t - 1]
if not node_to_split.leaf:
new_node.children = node_to_split.children[t:2 * t]
node_to_split.children = node_to_split.children[:t]
# Usage example
bptree = BPlusTree(3)
for key in [10, 20, 5, 6, 12, 30, 7, 17]:
bptree.insert(key)
Comparison and Analysis
The primary differences between B-trees and B+ trees lie in their structure and efficiency for particular operations:
Feature | B-Tree | B+ Tree |
---|---|---|
Data Storage | Data and keys stored in nodes | Data only stored in leaf nodes |
Space Efficiency | Less fan-out, taller trees | More fan-out, shorter trees |
Range Queries | Sequential traversals complicated | Range queries more efficient |
Insertion/Deletion Cost | Higher due to data reorganizing | Less due to only key reorganizing |
Complexity | Logarithmic | Logarithmic |
Additional Resources and References
For those interested in delving deeper into the implementation and theoretical underpinnings of B-trees and B+ trees, the following resources are recommended:
- "Introduction to Algorithms" by Thomas H. Cormen et al.
- "Database System Concepts" by Avi Silberschatz, Henry Korth, and S. Sudarshan.
Online resources also include:
Understanding these structures can significantly enhance your ability to optimize queries within a DBMS, leading to more efficient data processing and retrieval.