3-tier Architecture
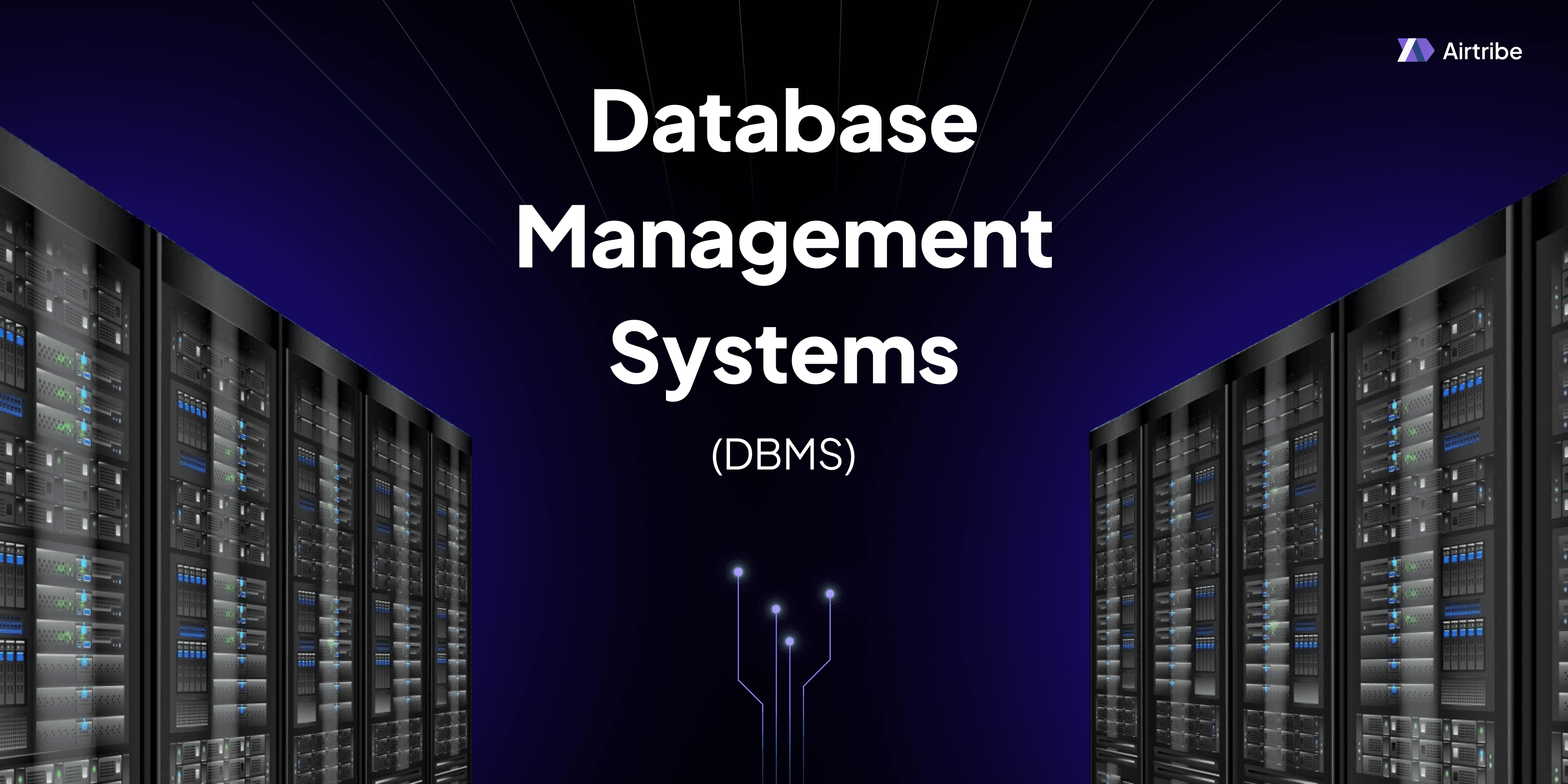
Understanding 3-Tier Architecture in Database Management Systems
In the realm of Database Management Systems (DBMS), architecture plays a pivotal role in how systems are structured, interact, and deliver results. One of the most prevalent architectures within this domain is the 3-tier architecture. This article delves into the 3-tier architecture, breaking down its components, core concepts, and its significance in DBMS.
Core Concepts and Theory
3-tier architecture separates a system into three distinct layers: the presentation layer, the application layer, and the data layer. This separation of concerns enhances scalability, maintainability, and supports distributed systems. Let's explore each tier in detail:
Presentation Layer: This is the user interface layer. It’s responsible for displaying data to and collecting input from users. Technologies used here include HTML, CSS, JavaScript, and frameworks like React or Angular.
Application Layer (Business Logic Layer): Also known as the middle tier, this layer processes the business logic underlying the application's requirements. It acts as a conduit between the user interface and the data layer. Here, programming languages such as Java, C#, or PHP are commonly deployed, alongside frameworks like Spring Boot or ASP.NET.
Data Layer (Database Layer): The third tier is where data is stored, retrieved, and managed. It includes the database server, responsible for data persistence and management. This layer commonly involves relational databases such as MySQL, PostgreSQL, or Oracle DB.
Each layer operates independently yet interacts symbiotically to create a robust and flexible framework enabling efficient data management and system functionality.
Practical Applications
3-tier architecture is renowned for its versatility and is employed extensively across various industries and applications. Here’s why it’s often favored:
- Scalability: Each layer can be scaled independently depending on the workload or traffic, offering a flexible way to handle growth.
- Maintainability: Maintenance becomes more efficient as changes in one layer have minimal impact on others, fostering easier management of updates and fixes.
- Security: Separation into distinct layers allows for more granular security measures, especially critical in the data layer where sensitive information resides.
Code Implementation and Demonstrations
Consider a simplified implementation scenario involving a web application demonstrating how a 3-tier architecture is structured. The example leverages a simple user management system.
Presentation Layer Example using HTML:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>User Management</title>
</head>
<body>
<h1>User Login</h1>
<form action="/login" method="post">
<label for="username">Username:</label>
<input type="text" id="username" name="username">
<br>
<label for="password">Password:</label>
<input type="password" id="password" name="password">
<br>
<button type="submit">Login</button>
</form>
</body>
</html>
Application Layer using Node.js:
const express = require('express');
const app = express();
app.post('/login', (req, res) => {
// Business logic for user authentication
// Interacts with Data Layer to verify credentials
res.send('User login handled in the application layer');
});
app.listen(3000, () => {
console.log('Server running on port 3000');
});
Data Layer using SQL:
CREATE TABLE Users (
ID SERIAL PRIMARY KEY,
Username VARCHAR(50) NOT NULL,
PasswordHash VARCHAR(255) NOT NULL
);
-- SQL Query to authenticate user
SELECT * FROM Users WHERE Username = 'username' AND PasswordHash = 'somehash';
Comparison and Analysis
3-tier vs. Other Architectures:
- 1-tier Architecture: Also known as monolithic, all components are tightly integrated. This simplicity can limit scalability and flexibility.
- 2-tier Architecture: Entails a client and server architecture. While it provides more separation than 1-tier, it lacks the middle layer for business logic, which could hinder scalability and security compared to 3-tier architecture.
Architecture | Layers | Pros | Cons |
---|---|---|---|
1-tier (Monolithic) | Single Layer | Simple to develop and deploy | Limited scalability and reusability |
2-tier | Client-Server | More separation than 1-tier, faster development | Less flexible, less scalable than 3-tier |
3-tier | Three Layers | Highly scalable, easy maintenance, robust security | More complex setup |
Additional Resources and References
- Books: "Designing Data-Intensive Applications" by Martin Kleppmann
- Online Courses: "Database Management Essentials" on Coursera
- Websites: TutorialsPoint’s DBMS sections, W3Schools
For further understanding, exploring these resources can offer deeper insights into the architectural paradigms that govern modern databases and applications. Learning and mastering 3-tier architecture empowers SDEs to build scalable, reliable, and maintainable systems, crucial in today's technology-driven landscape.