Error detection and correction
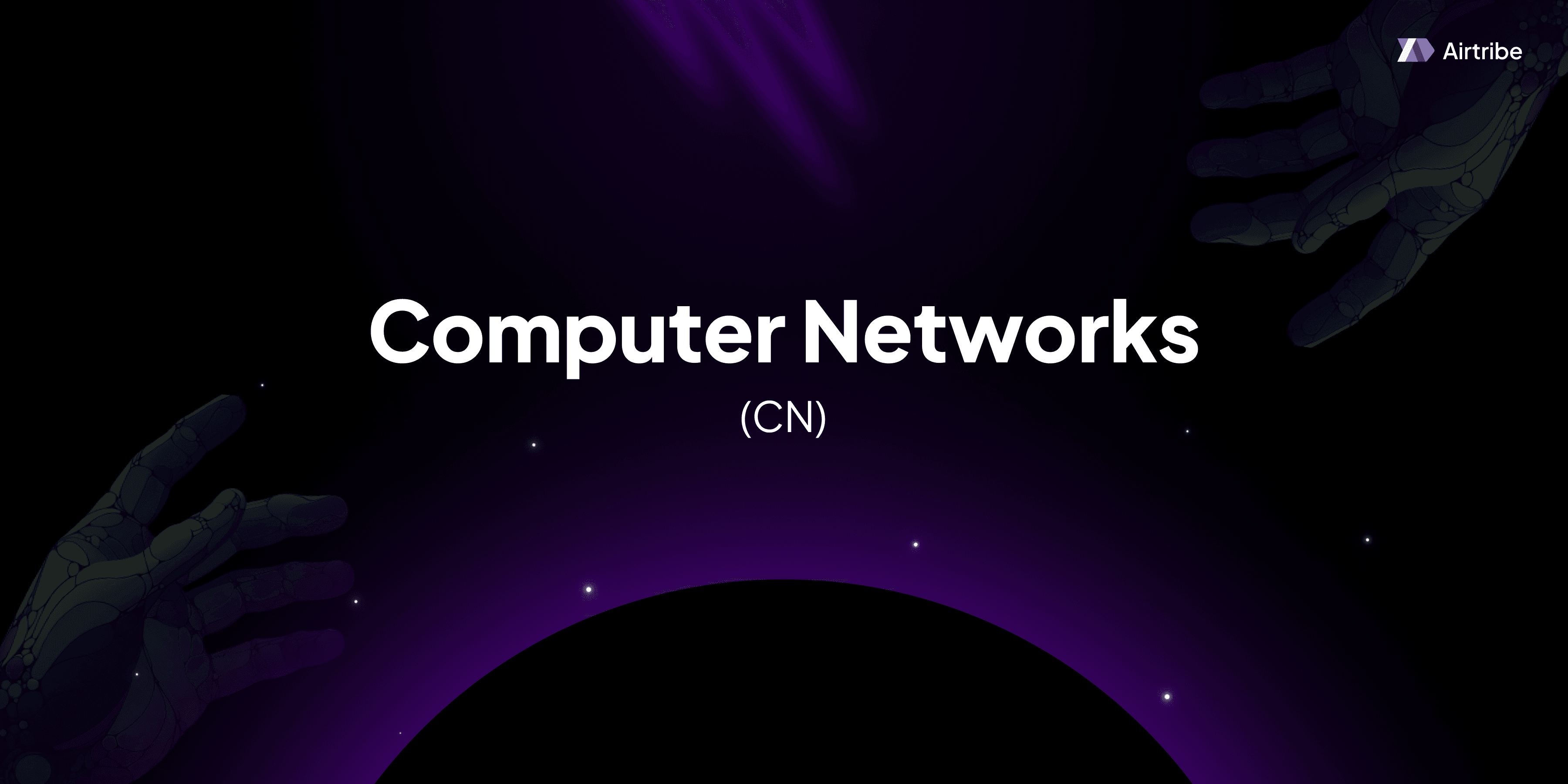
Understanding Error Detection and Correction
In computer networks, ensuring data integrity during transmission is crucial. As data travels across various mediums and through different network layers, it encounters numerous challenges, such as noise, interference, and signal attenuation. These challenges can lead to errors in the data. The data link layer, which is responsible for node-to-node data transfer, plays a significant role in error detection and correction, allowing robust communication over potentially unreliable physical connections.
Core Concepts and Theory
Error Types
Single-bit Errors: These occur when one bit of a data packet is altered during transmission. Single-bit errors can be caused by random electrical interference.
Burst Errors: These involve two or more bits in the data packet being altered, also known as packet errors. They are often caused by more significant disturbances in the transmission medium.
Error Detection Techniques
To detect errors, the data link layer uses several techniques:
Parity Checks: Involves adding an extra bit, known as a parity bit, to data. It can be either even or odd parity, ensuring the total number of 1-bits is even or odd. It's simple but not very robust against burst errors.
Checksums: Utilizes the sum of all data units to detect changes. The sender computes a checksum value and appends it to the data. The receiver recalculates the checksum to verify integrity.
Cyclic Redundancy Check (CRC): Incorporates polynomial division to detect errors. This method is effective in detecting burst errors and is widely used for its robustness and efficiency.
Error Correction Techniques
Forward Error Correction (FEC): The sender encodes redundant data with the original data signal, allowing the receiver to detect and correct errors without needing retransmission. Common FEC codes include Hamming Code and Reed-Solomon.
Automatic Repeat reQuest (ARQ): Focuses on error detection using acknowledgments and timeouts to request retransmission of erroneous data. Variants include Stop-and-Wait ARQ, Go-Back-N ARQ, and Selective Repeat ARQ.
Error Correction Codes
Hamming Code: Designed for correcting single-bit errors and detecting two-bit errors. It uses redundant bits placed at strategic positions within the data to check for consistency.
Reed-Solomon Code: An advanced error correction method widely used in digital communication and storage. It can correct multiple symbol errors and is suitable for bursts of data corruption.
Practical Applications
In practical settings, error detection and correction mechanisms are utilized extensively across various technologies:
Communication Systems: From satellite transmissions to terrestrial radio, error correction ensures accuracy in data delivery.
Storage Devices: Hard drives and SSDs use error correction to maintain data integrity against corruption over time due to wear and other factors.
Digital Media: CDs, DVDs, and Blu-rays employ error correction to handle scratches and read errors during playback.
Code Implementation and Demonstrations
To illustrate the concept of error detection and correction, let's explore a simple implementation of the Hamming Code in Python:
def calculate_parity_bits(data_bits):
n = len(data_bits)
i = 0
position = 0
while (2 ** i) < n + i + 1:
start_idx = (2 ** i) - 1
count = 0
while start_idx < n:
block = data_bits[start_idx: start_idx + (2 ** i)]
count += sum(int(bit) for bit in block)
start_idx += 2 * (2 ** i)
position += (count % 2) * (10 ** i)
i += 1
return position
def hamming_encode(data_bits):
n = len(data_bits)
m = 0
while (2 ** m) < n + m + 1:
m += 1
hamming_data = ['0'] * (n + m)
j = 0
k = 0
for i in range(len(hamming_data)):
if (math.log(i + 1, 2)).is_integer():
hamming_data[i] = 'p' # Placeholders for parity bits
else:
hamming_data[i] = data_bits[j]
j += 1
parity_bits = calculate_parity_bits(hamming_data)
for i in range(m):
if parity_bits % 10 != 0:
hamming_data[(2 ** i) - 1] = str(parity_bits % 10)
parity_bits //= 10
return ''.join(hamming_data)
data = "1011"
encoded_data = hamming_encode(data)
print(f"Encoded data with Hamming Code: {encoded_data}")
Comparison and Analysis
Efficiency: While parity checks are simple and easy to implement, they are limited in error detection capabilities. CRC is more efficient for detecting burst errors, and FEC techniques allow error correction without retransmission.
Complexity: FEC techniques like Hamming and Reed-Solomon are computationally complex but can correct errors in real time, providing resilience in dynamic environments.
Use Case Suitability: The choice among these techniques often depends on the application requirement for accuracy versus computational overhead. For instance, ARQ is suitable for reliable communication, while FEC is optimal for one-way communication scenarios.
Additional Resources and References
RFC 3385: Enhancing TCP Over Satellite Channels Using Standard Mechanisms
"Data and Computer Communications" by William Stallings provides a comprehensive overview of data transmission concepts, including error handling methods.
In summary, the data link layer's emphasis on error detection and correction is pivotal for maintaining data integrity and ensures reliable communication across diverse network conditions. These mechanisms are fundamental in a wide variety of applications, from storage to streaming, making them essential knowledge for anyone working in computer networks.