Diameter of Binary Tree
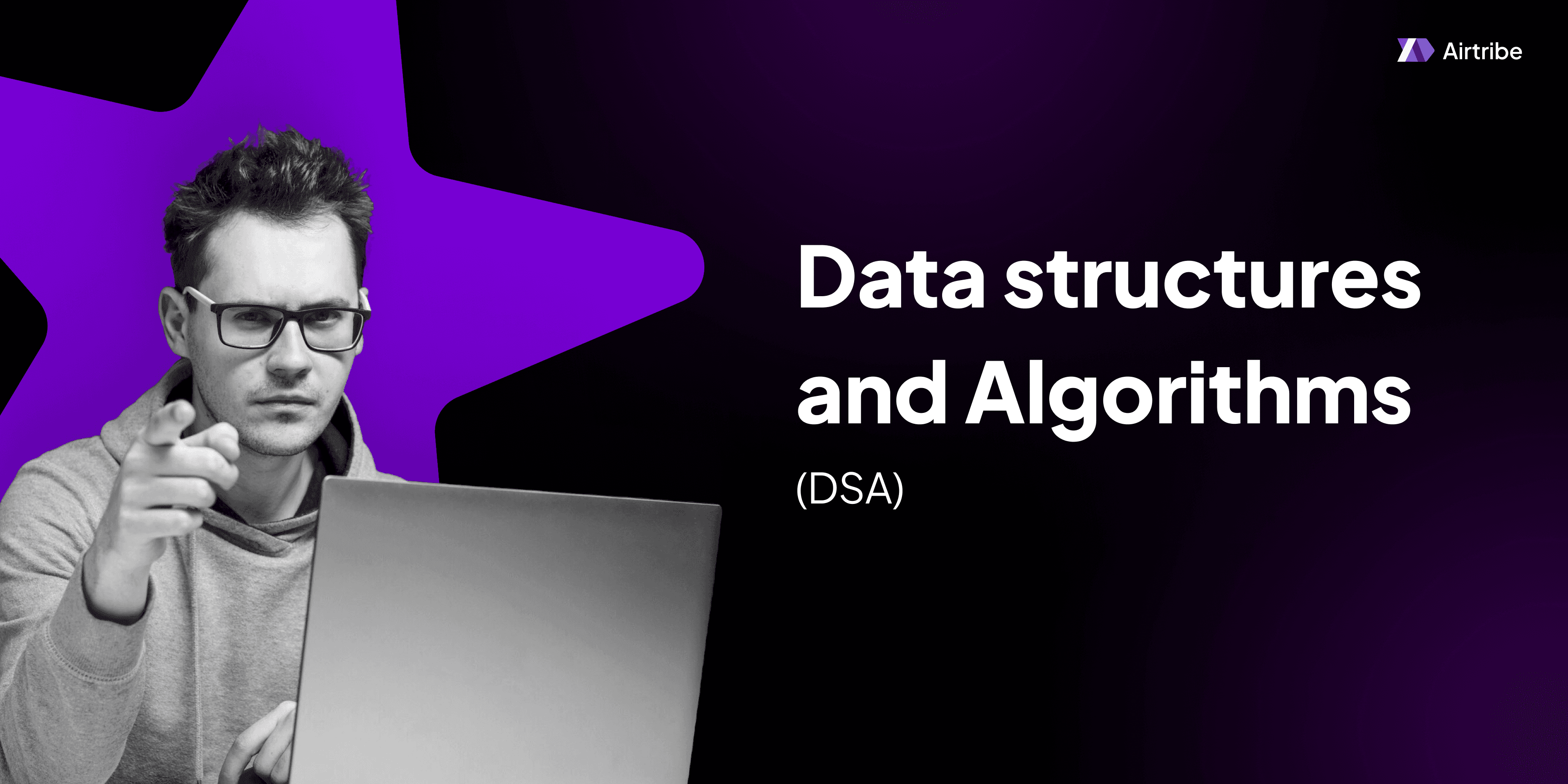
Problem Overview
The "Diameter of Binary Tree" problem is a popular coding challenge found on LeetCode, where the goal is to determine the longest path between any two nodes in a given binary tree. The path may or may not pass through the root of the tree, and it involves counting the number of edges in this path.
Understanding the Problem
Before delving into the solution, let's break down what the problem statement means. A binary tree is a tree data structure in which each node has at most two children referred to as the left child and the right child. The diameter of a binary tree is defined as the longest path between any two nodes, measured in terms of the total number of edges in the path.
Key DSA Concepts and Theory
Understanding this problem relies heavily on knowledge of binary trees, recursion, and depth-first search (DFS).
Binary Tree
A binary tree is a hierarchical structure with a root node and at most two child nodes for every parent node. Nodes at the same hierarchical level of the tree can be siblings if they share the same parent node.
Depth-First Search (DFS)
DFS is a common algorithm used for traversing or searching tree or graph data structures. The algorithm starts at the root node (selecting a node as the root in the graph case) and explores as far as possible along each branch before backtracking.
Recursion
Recursion is a common method for solving problems where the solution involves solving smaller instances of the same problem. In tree problems, recursion helps in elegantly breaking down the tree into smaller sub-trees.
Solution Approach
To solve the Diameter of Binary Tree problem, we can use a recursive approach to traverse the tree. The main idea is to perform a DFS while maintaining the depth of each subtree to calculate the potential path that gives the maximum diameter.
Detailed Steps
Define a Recursive Function: Create a helper function that computes the height of a tree and simultaneously updates the diameter variable to keep track of the maximum diameter found so far.
Base Case: If a node is
null
, the height is0
.Recursive Case: For each node, compute the height of its left and right subtrees.
Update Diameter: The potential diameter at each node will be the sum of the height of the left subtree and the right subtree. Update the diameter if the current potential diameter exceeds the existing diameter.
Height Calculation: Return the height of the tree rooted at the current node. The height of a node is the max height of its left or right subtree plus 1 for the current node.
Result: After the traversal, the diameter will be stored in a global or member variable.
Code Example: C++
class Solution {
public:
int diameterOfBinaryTree(TreeNode* root) {
int diameter = 0;
dfs(root, diameter);
return diameter;
}
private:
int dfs(TreeNode* node, int& diameter) {
if (!node) return 0;
int leftHeight = dfs(node->left, diameter);
int rightHeight = dfs(node->right, diameter);
diameter = std::max(diameter, leftHeight + rightHeight);
return std::max(leftHeight, rightHeight) + 1;
}
};
Code Example: Java
class Solution {
private int diameter = 0;
public int diameterOfBinaryTree(TreeNode root) {
dfs(root);
return diameter;
}
private int dfs(TreeNode node) {
if (node == null) return 0;
int leftHeight = dfs(node.left);
int rightHeight = dfs(node.right);
diameter = Math.max(diameter, leftHeight + rightHeight);
return Math.max(leftHeight, rightHeight) + 1;
}
}
Time and Space Complexity Analysis
Time Complexity: The algorithm runs in O(N) time, where N is the number of nodes in the tree. This is because we visit each node once during the DFS traversal.
Space Complexity: The space complexity is O(H), where H is the height of the tree. This is due to the recursive call stack. In the worst case, H can be N (in a completely unbalanced tree like a linked list).
Common Mistakes to Avoid
- Confusing Depth and Diameter: Ensure that you recognize the diameter is measured in edges, not nodes.
- Properly Updating Diameter: Make sure to update the diameter before returning the height from the recursive function.
Similar Problems on LeetCode
- Longest Path With Different Adjacent Characters - LeetCode #2487
- Maximum Depth of Binary Tree - LeetCode #104
- Balanced Binary Tree - LeetCode #110
Additional Resources and References
- LeetCode Discuss - Diameter of Binary Tree
- GeeksforGeeks - Diameter of a Binary Tree
- Introduction to Algorithms by Cormen - Trees
By understanding the problem and following the outlined steps, one can effectively solve the "Diameter of Binary Tree" challenge on LeetCode and gain deeper insights into tree traversal algorithms and their applications.